The Payments API
Onymos Payments is a drop-in solution for in-app purchases and payments. It’s fully integrated with the most popular payment gateways and services — right off-the-shelf.
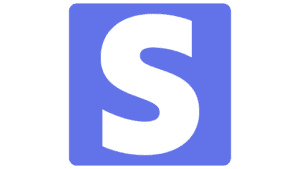
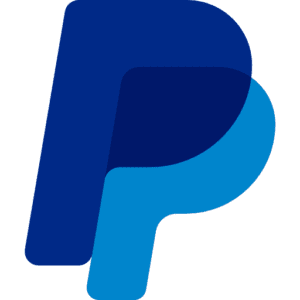
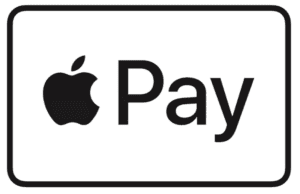
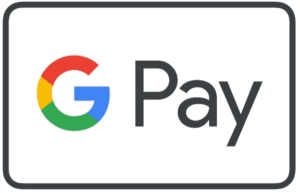
Using Payments
The Onymos Payments API provides a flexible and convenient range of normalized options for app users via direct, subscription, or marketplace payments.
OnymosPayments.cancelSubscription
Cancel a subscription with the specified provider.
function cancelSubscription() {
OnymosPayment.cancelSubscription(<subscriptionId>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
subscriptionId | String | The ID of the subscription to cancel |
OnymosPayments.canMakePayment
Checks ability or readiness to make a payment with the specified provider.
function canMakePayment() {
OnymosPayment.canMakePayment(<paymentObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
paymentObject = {
//required - string; 'stripe', 'paypal', 'apple', or 'google'
provider: '',
//optional - array<string> of payment methods, ex. 'credit'
paymentMethods: [],
//optional - array<string> of payment network names
paymentNetworks: []
}
OnymosPayments.getPaymentDetails
Get a summary of payments for a user.
function getPaymentDetails() {
OnymosPayment.getPaymentDetails(<userId>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the user to retrieve payments summary. |
OnymosPayments.getPayoutDetails
Get a summary of payouts for a user.
function getPayoutDetails() {
OnymosPayment.getPayoutDetails(<userId>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the user to retrieve payouts summary. |
OnymosPayments.getRegisteredAccounts
Get all payout account information registered for the user.
function displayUserPaymentAccounts() {
OnymosPayment.getRegisteredAccounts(<userId>)
.then((paymentAccounts) => {
console.log(paymentAccounts);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the user to retrieve accounts. |
OnymosPayments.getSubscription
Get the details of a subscription
function getSubscription() {
OnymosPayment.getSubscription(<subscriptionId>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
subscriptionId | String | The ID of the subscriptions |
OnymosPayments.listSubscriptions
List a customer’s subscriptions
function listSubscriptions() {
OnymosPayment.listSubscriptions(<customerId>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
customerId | String | The ID of the customer to show subscriptions |
OnymosPayments.makePayment
Initiate payment process with the specified provider.
function makePayment() {
OnymosPayment.makePayment(<paymentObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
paymentObject = {
//required - string; must be either 'stripe', 'paypal', 'apple', or 'google'
provider: '',
//required - string;
currency: 'USD';
//required - string;
countryCode: 'USD';
//required - array of payment items with price
purchaseItems: [
{
//required - number
price: 0,
//optional
label: ''
}
]
}
OnymosPayments.makeSubscriptionPayment
Initiate payment process for a subscription with the specified provider.
function makeSubscriptionPayment() {
OnymosPayment.makeSubscriptionPayment(<subscriptionObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
subscriptionObject = {
//required - string; must be either 'stripe', 'paypal', 'apple', or 'google'
provider: '',
//required - string;
currency: 'USD',
//required - string;
countryCode: 'USD',
//required - array of subscription items with price
subscriptionItems: [
{
//required - number
price: 0,
//optional
label: ''
}
],
//optional - number; the cost to start the subscription:
subscriptionSetupCost: 0,
// required - number; the start time of the subscription
startTime: 0,
// required - number; the end time of the subscription
endTime: 2,
// required - number; amount of days in the subscription interval
interval: 1,
}
OnymosPayments.payout
Send a payment to a user.
function sendPayout() {
OnymosPayment.payout(<userId>, <paymentObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the logged-in user. |
payoutObject = {
// provider - required, string; must be either 'paypal' or 'stripe'
provider: '',
// amount - required, string; amount of payment to be issued
amount: '',
// currency - required, string; valid entry 'USD'
currency: 'USD',
// emailSubject - optional, string; if not specified 'Payout' will
// be used as email subject
emailSubject: '',
}
OnymosPayments.registerAccount
Register a Paypal or Stripe payout account for a user.
function registerPaymentAccount() {
OnymosPayment.registerAccount(<userId>, <registerObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the logged-in user. |
registerObject = {
provider: 'stripe',
// email - required, string
email: '',
// fname - required, string; First Name
fname: '',
// lname - required, string; Last Name
lname: '',
// day - required, string; Day of Birth 1-31
day: '',
// month - required, string; Month of Birth 1-12
month: '',
// year - required, string; 4 digit Year of Birth
year: '',
// accountNumber - required, string; the Stripe account number
accountNumber: '',
// routingNumber - required, string; the routing number for the Stripe account
routingNumber: ''
}
OnymosPayments.removeAccount
Remove a payout account registered for the user.
function removeAccount() {
OnymosPayment.removeAccount(<userId>, <accountObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the user whose account is to be removed. |
accountObject = {
// provider - required, string; must be either 'stripe' or 'paypal'
provider: ''
}
OnymosPayments.setDefaultAccount
Set one of the ‘paypal’ or ‘stripe’ payout accounts already registered for the user as the default payout account.
function setDefaultAccount() {
OnymosPayment.setDefaultAccount(<userId>, <accountObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
userId | String | User ID of the user whose account is to be set as the default. |
accountObject = {
// provider - required, string; must be either 'stripe' or 'paypal'
provider: ''
}
OnymosPayments.updateSubscription
Initiate payment process for a subscription with the specified provider.
function updateSubscription() {
OnymosPayment.updateSubscription(<subscriptionId>, <subscriptionObject>)
.then((success) => {
console.log(success);
})
.catch((error) => {
console.log(error);
});
}
Key | Type | Description |
---|---|---|
subscriptionId | String | The ID of the subscription to update. |
subscriptionObject = {
//required - string; must be either 'stripe', 'paypal', 'apple', or 'google'
provider: '',
// optional - number; the end time of the subscription
endTime: 2,
// optional - number; the start time of the subscription
startTime: 0,
// optional - number; amount of days in the subscription interval
interval: 1,
// optional - array of subscription items;
subscriptionItems: [
{
//required - number
price: 0,
//optional
label: ''
}
],
//optional - string; description of the subscription
description: ''
// optional: string; the payment method
paymentMethod: ''
}